전체 소스코드
import tkinter.messagebox as msgbox
from tkinter import *
root = Tk()
root.title("GUI")
root.geometry("300x150")
#기차 예매 시스템이라고 가정
def info():
msgbox.showinfo("알림", "정상적으로 예매 완료되었습니다.")
def warn():
msgbox.showwarning("경고", "해당 좌석은 매진되었습니다.")
def error():
msgbox.showerror("에러", "결제 오류가 발생했습니다.")
def okCancel():
msgbox.askokcancel("확인 / 취소", "해당 좌석은 유아동반석입니다. 예매하시겠습니까?")
def retryCancel():
response = msgbox.askretrycancel("재시도 / 취소", "일시적인 오류입니다. 다시 시도하시겠습니까?")
if response == 1:
print("재시도")
elif response == 0:
print("취소")
def yesNo():
msgbox.askyesno("예 / 아니오", "해당 좌석은 역방향입니다. 예매하시겠습니까?")
def yesNoCancel():
response= msgbox.askyesnocancel(title=None, message="예매 내역이 저장되지 않았습니다.\n저장 후 프로그램을 종료하시겠습니까?")
#예: 저장 후 종료
#아니오: 저장하지 않고 종료
#취소: 프로그램 종료 취소(현재 화면에서 계속 작업)
print("응답:", response) #True(1), False(0), None(그 외)
if response == 1:
print("예")
elif response == 0:
print("아니오")
else:
print("취소")
Button(root, command=info, text="알림").pack()
Button(root, command=warn, text="경고").pack()
Button(root, command=error, text="에러").pack()
Button(root, command=okCancel, text="확인 / 취소").pack()
Button(root, command=retryCancel, text="재시도 / 취소").pack()
Button(root, command=yesNo, text="예 / 아니오").pack()
Button(root, command=yesNoCancel, text="예 / 아니오 / 취소").pack()
root.mainloop()
MessageBox 종류
메세지박스는 여러 종류가 있다
def info():
msgbox.showinfo("알림", "정상적으로 예매 완료되었습니다.")
.showinfo는 정보를 알려준다
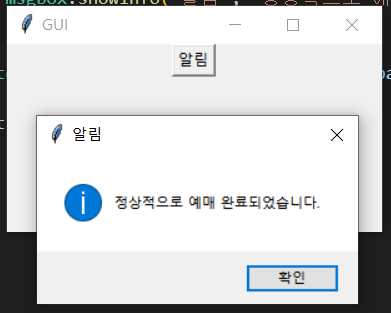
def warn():
msgbox.showwarning("경고", "해당 좌석은 매진되었습니다.")
.showwarning는 경고창을 띄워준다
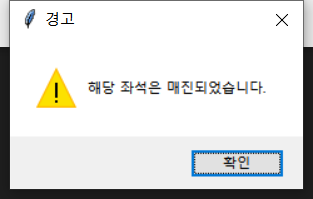
def error():
msgbox.showerror("에러", "결제 오류가 발생했습니다.")
.showerror는 에러창을 띄워준다
에러는 버튼을 클릭했을 때 나오는 경고음이 다르다
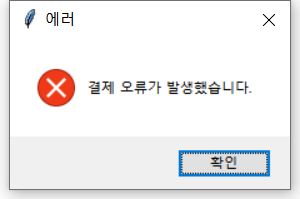
아래부터는 사용자의 의견을 묻는다
def okCancel():
msgbox.askokcancel("확인 / 취소", "해당 좌석은 유아동반석입니다. 예매하시겠습니까?")
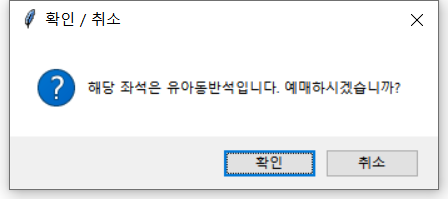
def retryCancel():
response = msgbox.askretrycancel("재시도 / 취소", "일시적인 오류입니다. 다시 시도하시겠습니까?")
if response == 1:
print("재시도")
elif response == 0:
print("취소")
.askretrycancel은 사용자가 다시 시도를 누른다면 1, 취소를 누른다면 0의 값을 반환한다
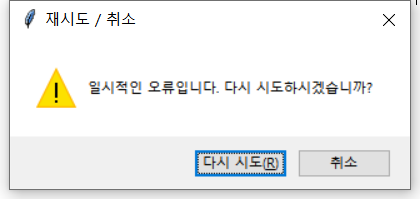
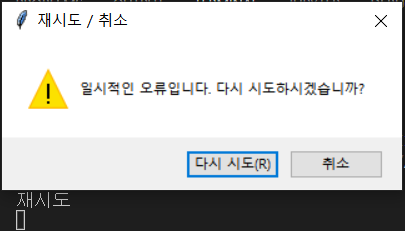
def yesNo():
msgbox.askyesno("예 / 아니오", "해당 좌석은 역방향입니다. 예매하시겠습니까?")
.askyesno는 취소 선택지가 없어서 엑스버튼이 눌리지 않는다
나오려면 아니요를 눌러 창을 빠져나와야한다
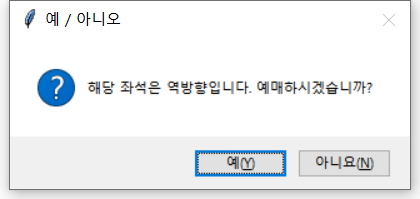
def yesNoCancel():
response= msgbox.askyesnocancel(title=None, message="예매 내역이 저장되지 않았습니다.\n저장 후 프로그램을 종료하시겠습니까?")
#예: 저장 후 종료
#아니오: 저장하지 않고 종료
#취소: 프로그램 종료 취소(현재 화면에서 계속 작업)
print("응답:", response) #True(1), False(0), None(그 외)
if response == 1:
print("예")
elif response == 0:
print("아니오")
else:
print("취소")
.askyesnocancel는 사용자가 예를 누른다면 1, 아니요를 누른다면 0의 값을 반환한다
취소는 None이므로 else로 처리해주면 된다
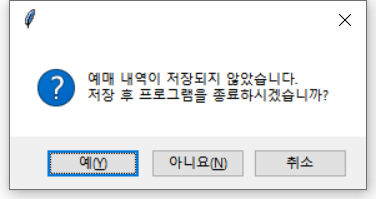
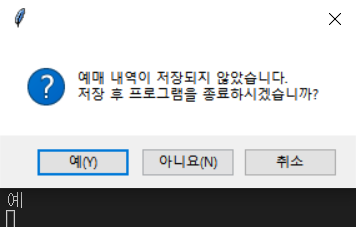
'python > tkinter' 카테고리의 다른 글
[Python tkinter GUI] #13 Scrollbar(스크롤바) (0) | 2022.12.16 |
---|---|
[Python tkinter GUI] #12 Frame(프레임) (0) | 2022.12.16 |
[Python tkinter GUI] #10 Menu(메뉴) (0) | 2022.12.16 |
[Python tkinter GUI] #9 Progessbar(진행바) (0) | 2022.12.16 |
[Python tkinter GUI] #8 Combobox(콤보박스) (0) | 2022.12.16 |
댓글