전체 소스코드
from tkinter import *
root = Tk()
root.title("GUI")
root.geometry("300x150")
#맨 윗줄
btn_f16 = Button(root, text="F16", width=5, height=2)
btn_f17 = Button(root, text="F17", width=5, height=2)
btn_f18 = Button(root, text="F18", width=5, height=2)
btn_f19 = Button(root, text="F19", width=5, height=2)
btn_f16.grid(row=0, column=0, sticky=N+E+W+S, padx=3, pady=3)
btn_f17.grid(row=0, column=1, sticky=N+E+W+S, padx=3, pady=3)
btn_f18.grid(row=0, column=2, sticky=N+E+W+S, padx=3, pady=3)
btn_f19.grid(row=0, column=3, sticky=N+E+W+S, padx=3, pady=3)
#clear줄
btn_clear = Button(root, text="clear", width=5, height=2)
btn_equal = Button(root, text="=", width=5, height=2)
btn_div = Button(root, text="/", width=5, height=2)
btn_mul = Button(root, text="*", width=5, height=2)
btn_clear.grid(row=1, column=0, sticky=N+E+W+S, padx=3, pady=3)
btn_equal.grid(row=1, column=1, sticky=N+E+W+S, padx=3, pady=3)
btn_div.grid(row=1, column=2, sticky=N+E+W+S, padx=3, pady=3)
btn_mul.grid(row=1, column=3, sticky=N+E+W+S, padx=3, pady=3)
#7 시작 줄
btn_7 = Button(root, text="7", width=5, height=2)
btn_8 = Button(root, text="8", width=5, height=2)
btn_9 = Button(root, text="9", width=5, height=2)
btn_sub = Button(root, text="-", width=5, height=2)
btn_7.grid(row=2, column=0, sticky=N+E+W+S, padx=3, pady=3)
btn_8.grid(row=2, column=1, sticky=N+E+W+S, padx=3, pady=3)
btn_9.grid(row=2, column=2, sticky=N+E+W+S, padx=3, pady=3)
btn_sub.grid(row=2, column=3, sticky=N+E+W+S, padx=3, pady=3)
#4 시작 줄
btn_4 = Button(root, text="4", width=5, height=2)
btn_5 = Button(root, text="5", width=5, height=2)
btn_6 = Button(root, text="6", width=5, height=2)
btn_add = Button(root, text="+", width=5, height=2)
btn_4.grid(row=3, column=0, sticky=N+E+W+S, padx=3, pady=3)
btn_5.grid(row=3, column=1, sticky=N+E+W+S, padx=3, pady=3)
btn_6.grid(row=3, column=2, sticky=N+E+W+S, padx=3, pady=3)
btn_add.grid(row=3, column=3, sticky=N+E+W+S, padx=3, pady=3)
#4 시작 줄
btn_1 = Button(root, text="1", width=5, height=2)
btn_2 = Button(root, text="2", width=5, height=2)
btn_3 = Button(root, text="3", width=5, height=2)
btn_enter = Button(root, text="enter", width=5, height=2)
btn_1.grid(row=4, column=0, sticky=N+E+W+S, padx=3, pady=3)
btn_2.grid(row=4, column=1, sticky=N+E+W+S, padx=3, pady=3)
btn_3.grid(row=4, column=2, sticky=N+E+W+S, padx=3, pady=3)
btn_enter.grid(row=4, column=3, rowspan=2, sticky=N+E+W+S, padx=3, pady=3)
#0 시작 줄
btn_0 = Button(root, text="0", width=5, height=2)
btn_ponit = Button(root, text=".", width=5, height=2)
btn_0.grid(row=5, column=0, columnspan=2, sticky=N+E+W+S, padx=3, pady=3)
btn_ponit.grid(row=5, column=2, sticky=N+E+W+S, padx=3, pady=3)
root.mainloop()
Grid
btn1 = Button(root, text="버튼1")
btn2 = Button(root, text="버튼2")
btn1.pack()
btn2.pack()
btn1.grid(row=0, column=0)
btn2.grid(row=1, column=1)
좌표기반으로 위젯을 배치할 수 있다
pack은 쌓는 느낌
grid는 배치하는 느낌
row와 column으로 좌표를 설정할 수 있다
그리드의 전체 크기를 먼저 정해놓는다기보다는
row와 column을 정하면 그에 맞춰서 그리드를 그리는 것이 맞다
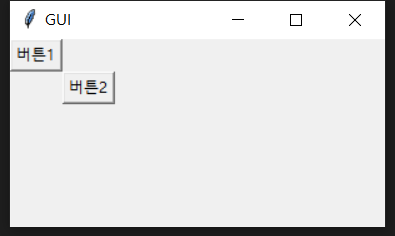
#맨 윗줄
btn_f16 = Button(root, text="F16", width=5, height=2)
btn_f17 = Button(root, text="F17", width=5, height=2)
btn_f18 = Button(root, text="F18", width=5, height=2)
btn_f19 = Button(root, text="F19", width=5, height=2)
btn_f16.grid(row=0, column=0, sticky=N+E+W+S, padx=3, pady=3)
btn_f17.grid(row=0, column=1, sticky=N+E+W+S, padx=3, pady=3)
btn_f18.grid(row=0, column=2, sticky=N+E+W+S, padx=3, pady=3)
btn_f19.grid(row=0, column=3, sticky=N+E+W+S, padx=3, pady=3)
#clear줄
btn_clear = Button(root, text="clear", width=5, height=2)
btn_equal = Button(root, text="=", width=5, height=2)
btn_div = Button(root, text="/", width=5, height=2)
btn_mul = Button(root, text="*", width=5, height=2)
btn_clear.grid(row=1, column=0, sticky=N+E+W+S, padx=3, pady=3)
btn_equal.grid(row=1, column=1, sticky=N+E+W+S, padx=3, pady=3)
btn_div.grid(row=1, column=2, sticky=N+E+W+S, padx=3, pady=3)
btn_mul.grid(row=1, column=3, sticky=N+E+W+S, padx=3, pady=3)
#7 시작 줄
btn_7 = Button(root, text="7", width=5, height=2)
btn_8 = Button(root, text="8", width=5, height=2)
btn_9 = Button(root, text="9", width=5, height=2)
btn_sub = Button(root, text="-", width=5, height=2)
btn_7.grid(row=2, column=0, sticky=N+E+W+S, padx=3, pady=3)
btn_8.grid(row=2, column=1, sticky=N+E+W+S, padx=3, pady=3)
btn_9.grid(row=2, column=2, sticky=N+E+W+S, padx=3, pady=3)
btn_sub.grid(row=2, column=3, sticky=N+E+W+S, padx=3, pady=3)
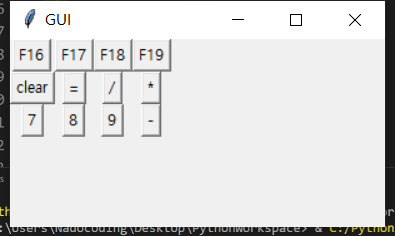
#4 시작 줄
btn_1 = Button(root, text="1", width=5, height=2)
btn_2 = Button(root, text="2", width=5, height=2)
btn_3 = Button(root, text="3", width=5, height=2)
btn_enter = Button(root, text="enter", width=5, height=2)
btn_1.grid(row=4, column=0, sticky=N+E+W+S, padx=3, pady=3)
btn_2.grid(row=4, column=1, sticky=N+E+W+S, padx=3, pady=3)
btn_3.grid(row=4, column=2, sticky=N+E+W+S, padx=3, pady=3)
btn_enter.grid(row=4, column=3, rowspan=2, sticky=N+E+W+S, padx=3, pady=3)
#0 시작 줄
btn_0 = Button(root, text="0", width=5, height=2)
btn_ponit = Button(root, text=".", width=5, height=2)
btn_0.grid(row=5, column=0, columnspan=2, sticky=N+E+W+S, padx=3, pady=3)
btn_ponit.grid(row=5, column=2, sticky=N+E+W+S, padx=3, pady=3)
rowspan
현재 위치로부터 n줄을 아래쪽으로 더한다
columnspan
현재 위치로부터 n줄을 오른쪽으로 더한다
그래서 실행결과처럼 enter는 가로 두 줄의 가운데에 위치하고
0은 세로 두 줄의 가운데에 위치한다
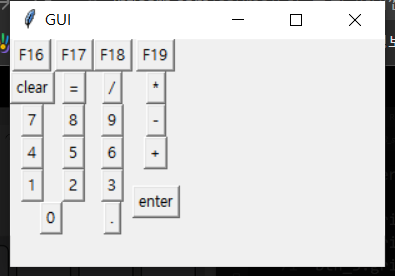
그러면 얼추 키보드의 숫자패드처럼 나온다
하지만 칸의 크기가 제각기여서 불완전하다
그래서 sticky라는 속성이 필요하다
NEWS로 지정할 수 있고 지정한 방향으로 다른 좌표와 달라붙는다
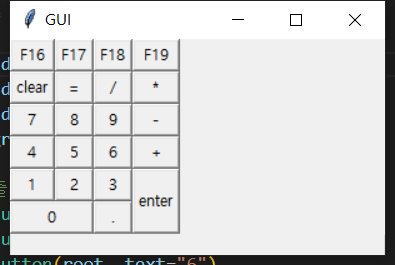
모든 키에 sticky 속성을 지정하니 정말 키보드 같아 보인다
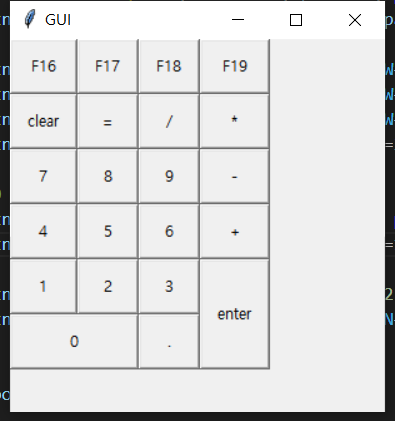
padx, pady로 공간을 넓혀주었다
grid에도 padx, pady 속성이 있다
하지만 버튼 안의 텍스트와 테두리 사이를 조정하는 버튼과 달리
그리드는 위젯 밖의 테두리와 다른 그리드 사이의 공간을 지정한다
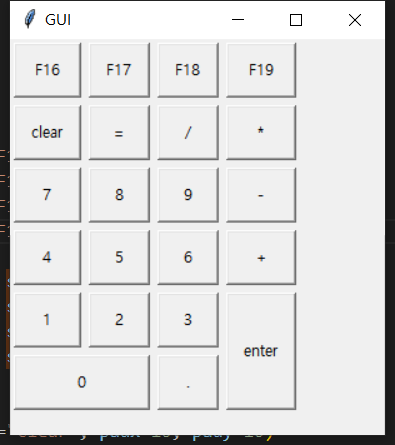
그럼 이렇게 공간이 생긴다
하지만 버튼 안의 텍스트를 기준으로 여백을 지정하다보니
버튼의 크기가 일정하지 않다
이 때 width, height를 이용하면 된다

그러면 안에 들어있는 텍스트와 상관없이 모든 버튼의 크기가 일정해진다
'python > tkinter' 카테고리의 다른 글
[Python tkinter GUI] #13 Scrollbar(스크롤바) (0) | 2022.12.16 |
---|---|
[Python tkinter GUI] #12 Frame(프레임) (0) | 2022.12.16 |
[Python tkinter GUI] #11 MessageBox(메세지박스) (0) | 2022.12.16 |
[Python tkinter GUI] #10 Menu(메뉴) (0) | 2022.12.16 |
[Python tkinter GUI] #9 Progessbar(진행바) (0) | 2022.12.16 |
댓글